みなさんこんにちは!ひろぽんです。
今回はInterfaceを継承しているかの判断をしたうえで、ついでにコンバートしてみるという題で書いていきたいと思います。
では早速!
目次
まずはこんなクラスを用意する!
Interfaceの準備
まずはInterfaceを二つ用意します!
public interface IVehicle
{
string MoveSound { get; }
}
public interface ICar
{
string Maker { get; }
}
でこれらを継承したクラスを用意します!
public class Subaru : IVehicle , ICar
{
public string MoveSound => "ブーブー";
public string Maker => "Subaru";
}
public class Plane : IVehicle
{
public string MoveSound => "ごぉーーーーーー";
}
SubaruクラスはIVehicle InterfaceとICar Interfaceを継承しています
が、PlaneクラスはIVehicle Interfaceしか継承していません!
Interfaceを継承しているかの判断
で、下記のようなコードを書いて、クラスがIntefaceを継承しているかを見てみましょう!
まずは下記のコードで継承しているかの判断ができます。
static void Main(string[] args)
{
var plane = new Plane();
var car = plane as ICar;
if (car != null)
{
Console.WriteLine(car.Maker);
}
else
{
Console.WriteLine("PlaneはICarを継承していません。");
}
Console.ReadLine();
}
継承していればNullにならずに変数にコンバートしたものがはいります。
が、継承していなければNullが入ります。
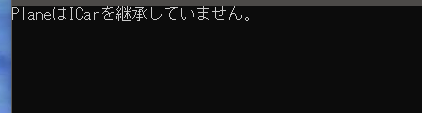
InterfaceからInterfaceの継承チェックもできる。
上記はクラスがInterfaceを継承しているかを見ましたが、Intefaceの型で別のInterfaceチェックもできます。
static void Main(string[] args)
{
IVehicle plane = new Plane();
var car = plane as ICar;
if (car != null)
{
Console.WriteLine(car.Maker);
}
else
{
Console.WriteLine("PlaneはICarを継承していません。");
}
Console.ReadLine();
}
}
こんな感じでvarからIVehicleにしてもチェックできますし、下記の感じでもできます。
static void Main(string[] args)
{
IVehicle subaru = new Subaru();
var car = subaru as ICar;
if (car != null)
{
Console.WriteLine(car.Maker);
}
else
{
Console.WriteLine("PlaneはICarを継承していません。");
}
Console.ReadLine();
}
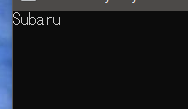
Interfaceを継承していたらコンバートする
とはいえ、一度変換した後にNullチェックをするのはめんどくさい!ということで、if分の中で同時に変換できます!
static void Main(string[] args)
{
IVehicle subaru = new Subaru();
if (subaru is ICar car)
{
Console.WriteLine(car.Maker);
return;
}
Console.WriteLine("PlaneはICarを継承していません。");
Console.ReadLine();
}
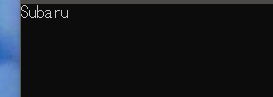
Planeクラスでやるとこんな感じになります。
static void Main(string[] args)
{
IVehicle plane = new Plane();
if (plane is ICar car)
{
Console.WriteLine(car.Maker);
Console.ReadLine();
return;
}
Console.WriteLine("PlaneはICarを継承していません。");
Console.ReadLine();
}
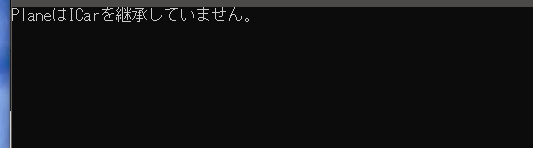
Githubで今回のコードを公開しています!
下記リポジトリのInterfaceConvertで今回のソースを公開しています!
GitHub

GitHub - HayashiyamaHiroshi/blog-source-demo
Contribute to HayashiyamaHiroshi/blog-source-demo development by creating an account on GitHub.
コメント